일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- 한번에끝내는JavaSpring웹개발마스터초격차패키지Online
- 웹
- 패스트캠퍼스
- 재택근무
- 자바기본
- javabasic
- 자바
- 직장인인강
- DB
- DesignPattern
- 직장인자기계발
- 디자인
- ncs
- 패캠챌린지
- java기초
- linux
- 자바기초
- 패스트캠퍼스후기
- 리눅스
- 자바연습문제
- String
- 데이터베이스
- 디자인패턴
- 국비
- js
- java
- 한번에끝내는JavaSpring웹개발마스터초격차패키지Online강의
- 자바예제
- Spring
- 스프링
- Today
- Total
FIF's 코딩팩토리
자바 기초 NCS교육과정(48)-쓰레드 본문
동기화 |
비동기화 |
StringBuilder |
StringBuffer |
Vector |
ArrayList |
Hashtable |
HashMap |
파일 : Ms-word프로그램
프로세스: 메모리에서 실행중인 프로그램이다.
게임프로그램-게임, 음악, 채팅
스레드(thread) : 프로세스에서 독립적으로 실행되는 단위이다.
자바에서 스레드 생성을 위해 제공해주는 2가지 방법
1. java.lang.Thread클래스
2. java.lang.Runnable 인터페이스
스케줄링
: 스레드가 생성되어 실행될 때 시스템의 여러 자원을 해당 스레드에게
할당하는 작업
선점형 스케줄링
:하나의 스레드가 cpu을 할당받아 실행하고 있을 때 우선순위가
높은 다른 스레드가 cpu를 강제로 빼앗아가 사용할 수 있는 스케줄링
기법이다.
Java206_thread.java
class User extends Thread{ public User() { } public void run() { //thread로 실행시켜줄 문장들은 run()메소드에서 구현한다. for(int i=0;i<=5;i++) { System.out.printf("%s i=%d\n",getName(),i); } } } public class Java206_thread { //실행 -> main스레드 -> main메소드 public static void main(String[] args) { User us=new User(); us.start();//2개 for(int j=0;j<=5;j++) { System.out.printf("%s j=%d\n",Thread.currentThread().getName(),j); } } }
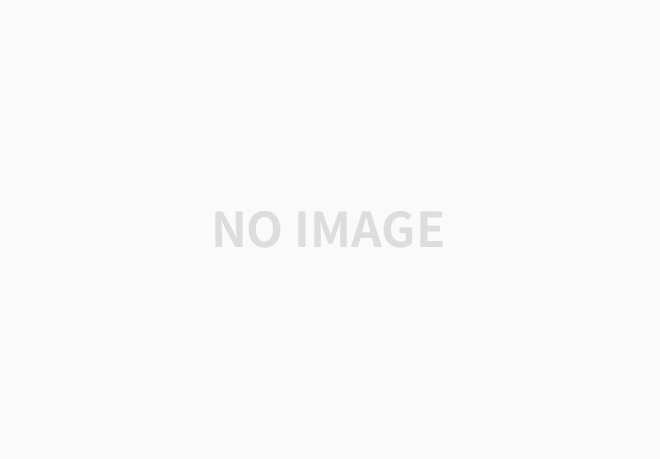
Java207_thread.java
class User2 implements Runnable{ public User2() { } @Override public void run() { for(int i=0;i<=5;i++) { System.out.printf("%s i=%d\n",Thread.currentThread().getName(),i); } } } public class Java207_thread { public static void main(String[] args) { User2 us=new User2(); new Thread(us).start(); Thread th=new Thread(us); th.start(); System.out.println("main thread"); } }
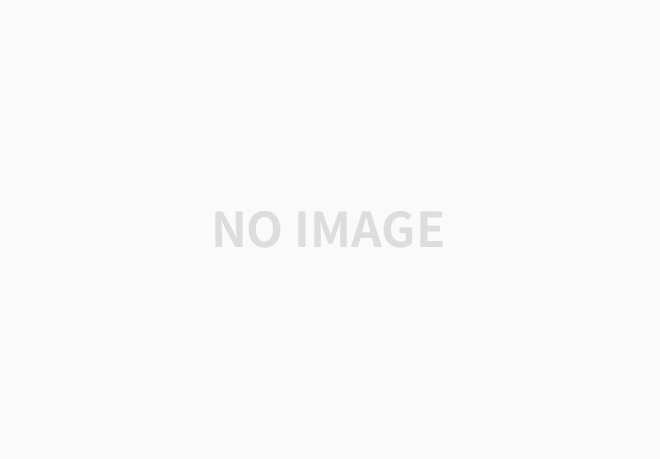
스레드 생명주기(Life Cycle)
start()-실행준비상태(RUNNABLE)-run()-TERMINATED-대기상태(WAITING, NOT RUNNABLE)
Java208_thread.java
class LifeCycle extends Thread{ public LifeCycle() { } @Override public void run() { System.out.println(getState());//스레드의 상태 for(int i=0;i<=5;i++) { System.out.printf("%s i=%d\n",getName(),i); try { //1000은 1초를 의미한다. Thread.sleep(1000);//WAITING(NOT RUNNABLE)- 일시정지상태 } catch (InterruptedException e) { e.printStackTrace(); } } } } public class Java208_thread { public static void main(String[] args) { LifeCycle cc= new LifeCycle(); System.out.println(cc.getState());//스레드의 상태 cc.start(); try { //지정된 시간동안 스레드가 실행되도록 한다. //지정된 시간이 지나거나 종료가 되면 join()을 호출함 //스레드로 다시 돌아와 실행을 계속 수행한다 cc.join(); } catch (InterruptedException e) { e.printStackTrace(); } System.out.println(cc.getState()); System.out.println("main end"); } }
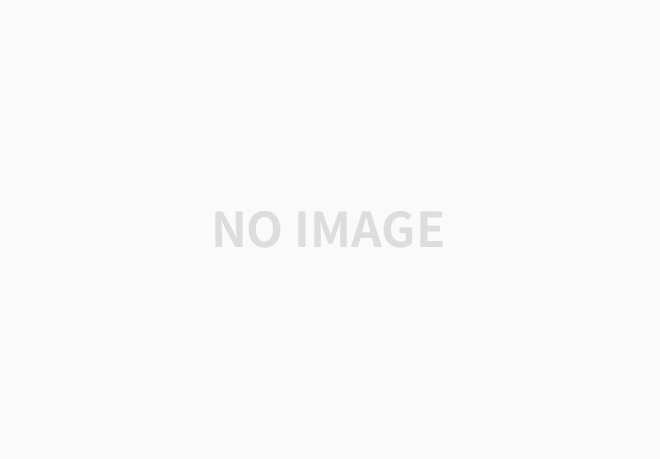
Java209_thread.java
class PriorityTest extends Thread{ @Override public void run() { for(int i=0;i<=5;i++) { System.out.printf("%s priority=%d i=%d\n",getName(),getPriority(),i); } } } public class Java209_thread { public static void main(String[] args) { PriorityTest t1= new PriorityTest(); t1.setName("user"); t1.start(); PriorityTest t2= new PriorityTest(); /* * 스레드의 우선순위는 1~10까지 지정할 수 있다. * 스레드의 기본값은 5이다. */ t2.setPriority(Thread.MAX_PRIORITY);//10 t2.start(); PriorityTest t3= new PriorityTest(); t3.setPriority(8); t3.start(); } }
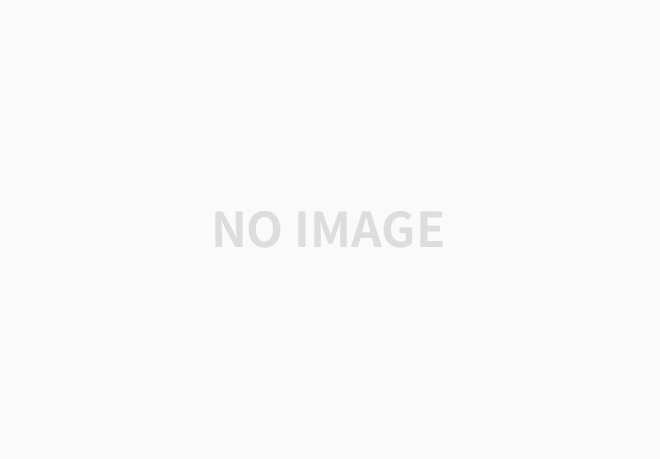
동기화(synchronized)
계좌번호 => 공유자원
A지점, B지점, C지점 => 스레드
동기화 : 하나의 스레드가 공유자원을 사용하고 있으면 다른 스레드가
접근하는 것을 막아주는 기능이다.
동기화 목적 : 데이터의 일관성 유지를 위해서이다.
동기화 키워드 : synchronized
동기화 설정방법
1) 메소드에 lock을 걸고자 할때
synchronized void openDoor(String name){}
2) 특정한 객체에 lock을 걸고자 할때
void openDoor(String name){
synchronized(객체의 참조변수){
}
}
wait(), notify(), notifyAll()메소드 동기화가
설정되여 있는 영역에서만 호출 할 수 있다.
Java210_thread.java
//공유자원 class Washroom { synchronized void openDoor(String name) { System.out.println(name + "님이 입장"); for (int i = 0; i < 50000; i++) { if (i % 10000 == 0) { try { Thread.sleep(1000); } catch (InterruptedException e) { e.printStackTrace(); } System.out.println(name + "님이 업무 보는중"); } } System.out.println(name + "님이 퇴장"); } }// end Washroom class FamilyThread extends Thread { private Washroom wr; private String who; public FamilyThread() { } public FamilyThread(Washroom wr, String who) { this.wr = wr; this.who = who; } @Override public void run() { wr.openDoor(who); } } public class Java210_thread { public static void main(String[] args) { Washroom wr = new Washroom(); FamilyThread father=new FamilyThread(wr, "father"); FamilyThread mother=new FamilyThread(wr, "mother"); FamilyThread sister=new FamilyThread(wr, "sister"); FamilyThread brother=new FamilyThread(wr, "brother"); father.start(); mother.start(); sister.start(); brother.start(); } }
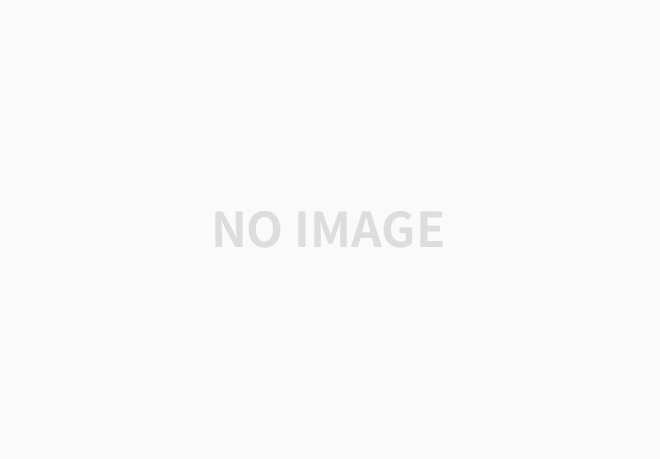
'Back-End > 국비 NCS교과과정' 카테고리의 다른 글
자바 기초 NCS교육과정(47)-inner (0) | 2019.08.06 |
---|---|
자바 기초 NCS교육과정(46)-컬렉션 문제풀이 (1) | 2019.08.02 |
자바 기초 NCS교육과정(45)-컬렉션4 (0) | 2019.08.02 |
자바 기초 NCS교육과정(44)-컬렉션3 (0) | 2019.08.02 |
자바 기초 NCS교육과정(43)-컬렉션2 (0) | 2019.08.02 |