일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- 직장인인강
- java기초
- 디자인
- js
- 직장인자기계발
- 디자인패턴
- java
- DesignPattern
- 자바연습문제
- 데이터베이스
- 국비
- String
- Spring
- 스프링
- 한번에끝내는JavaSpring웹개발마스터초격차패키지Online강의
- linux
- 패스트캠퍼스후기
- 자바기본
- 한번에끝내는JavaSpring웹개발마스터초격차패키지Online
- ncs
- javabasic
- 자바
- 패캠챌린지
- 웹
- 패스트캠퍼스
- DB
- 자바예제
- 자바기초
- 리눅스
- 재택근무
- Today
- Total
FIF's 코딩팩토리
자바 기초 NCS교육과정(18)-상속 본문
상속(inheritance) 이란? |
1 기본 클래스를 재사용하여 새로운 클래스를 정의하는 것이다.
2 두 클래스를 조상과 자손 관계를 맺어준다.(extends)
3 상속을 해주는 클래스 : 수퍼클래스, 부모클래스, 기본클래스
상속을 받는 클래스 : 서브클래스, 자식클래스, 유도클래스
4 자식(손)클래스는 조상의 모든 자원을 상속받는다.
(생성자, static{}은 제외)
5 자식(손)의 자원은 부모보다 크거나 같아야 한다.(자식클래스 >= 부모클래스)
6 상속을 할때 사용되는 키워드 : extends(단일상속)
class A{}
class B extend A{}
=>자식 클래스 B extends 부모클래스A
- A는 B에게 상속한다.
- B는 A이다.(상속관계의 클래스를 is a 관계라한다.)
class 도형{}
class 삼각형 extends 도형{}
삼각형은 도형이다.
class Test{}
class Sample{
public static void main(String[] args){
Test tt=new Test();
}
}
Sample은 Test를 포함한다.(포함관계-has a)
Java092_inheritance.java
class Father{ int a=3; void prn() { System.out.println("a="+a); } }//end Father //Child는 Father을 상속받는다(Child는 Father이다) class Child extends Father{ //Child 클래스는 Fatger클래스를 상속 받는다 int b=5; } public class Java092_inheritance { public static void main(String[] args) { Child cd=new Child(); System.out.println("cd.a="+cd.a); cd.prn(); } }
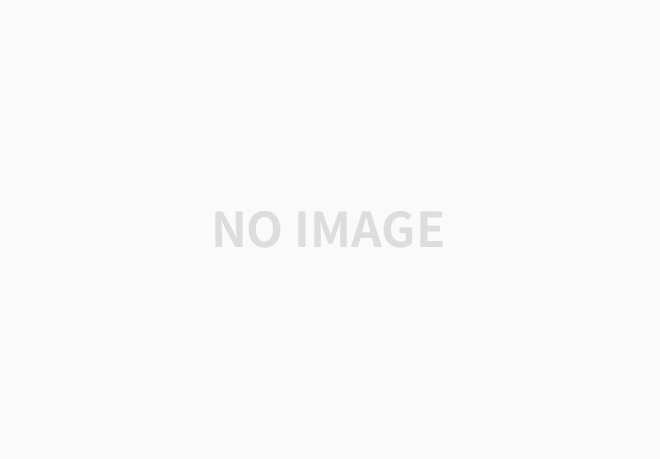
Java093_inheritance.java
class MyGrand /*extends Object*/ { public MyGrand() {//7 System.out.println("MyGrand");//8 }//9 } class MyFather extends MyGrand{ public MyFather() {//5 super();//6 System.out.println("MyFather");//10 } } class MyChild extends MyFather { public MyChild() {//3 //자식생성자에서는 반드시 부모생성자를 호출해야한다. //부모생성자 호출이 생략되어 있으면 JVM에서 super()로 호출한다. super();//부모객체 호출//4 System.out.println("MyChild");//11 }//12 } public class Java093_inheritance { public static void main(String[] args) {//1 MyChild m = new MyChild();//2 }//13 }
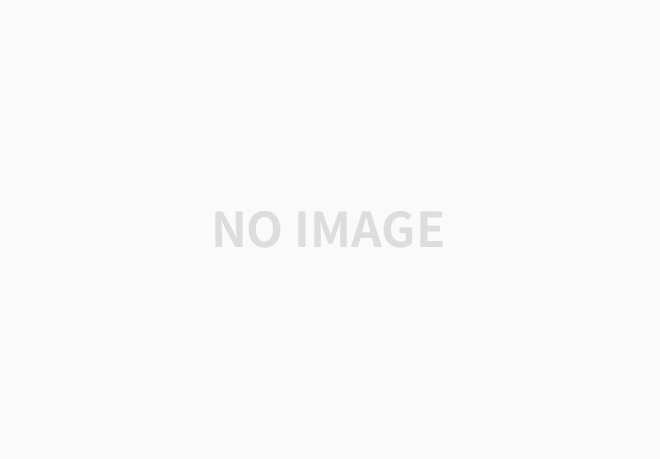
Java094_inheritance.java
class SuperConst{ int x; int y; public SuperConst(int x, int y) { this.x=x; this.y=y; } } class SubConst extends SuperConst{ public SubConst() { //super(); //SuperConst클래스에 1개의 생성자가 정의되여 있으므로 //JVM에서 기본생성자를 제공하지 않으므로 super()로 호출할 수 없다. super(10, 40); } } public class Java094_inheritance { public static void main(String[] args) { SubConst sc= new SubConst(); System.out.printf("x=%d y=%d\n",sc.x,sc.y); } }
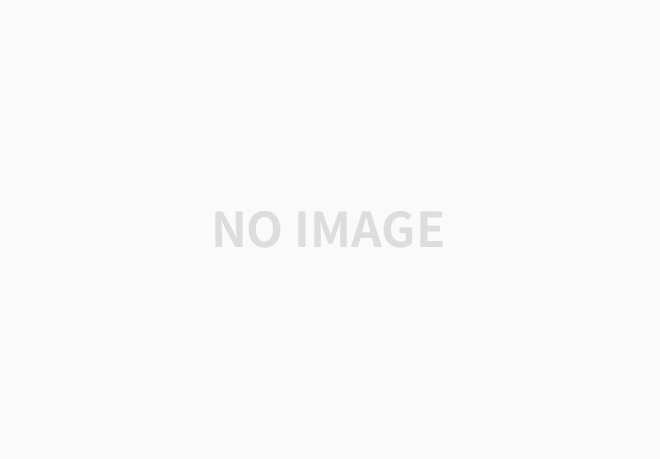
Java095_inheritance.java
class Parent{ String name; int age; public Parent() { } public Parent(String name, int age) {//5 this.name=name;//6 this.age=age;//7 }//8 } class Sun extends Parent{ String dept; public Sun() {} public Sun(String name, int age, String dept) {//3 ////////////////////// super(name, age);//4 this.dept=dept;//9 /////////////////////// }//10 public void prn() {//12 System.out.printf("%s %d %s\n",name,age,dept);//13 }//14 } public class Java095_inheritance { public static void main(String[] args) {//1 Sun ss=new Sun("홍길동",50,"기획부");//2 ss.prn();//11 }//15 }
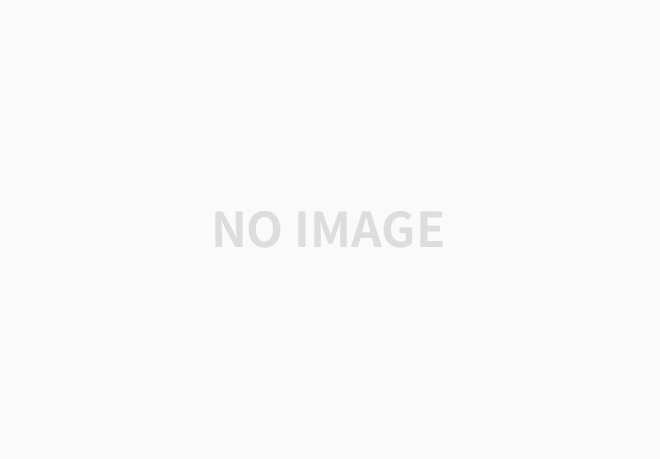
오버라이딩(overriding)
1 조상클래스의 메소드를 자식클래스에서 재정의(메소드 구현부)하는 기능이다.
2 메소드의 선언부는 그대로 사용한다.(리턴데이터타입, 메소드명, 매개변수)
단, 접근제어자는 같거나 크면된다.
private < default < protected < public
3 오버라이딩은 상속을 받은 후 할 수 있다.
super
1 자식클래스에서 부모클래스를 호출할때 사용한다.
2 super.멤버변수
super.메소드()
super() => 생성자
단일클래스 VS 상속클래스
overloading vs orverriding
this vs super
Java096_inheritance.java
class First { int a = 10; void prn() { System.out.println("a=" + a); } } class Second extends First { int b = 20; int a=30; @Override void prn() { System.out.printf("a=%d b=%d\n",super.a,b); } public void display() { System.out.println("display"); } public void superThisMethod() { super.prn();//static 에선 사용불가 this.prn();//static 에선 사용불가 } } public class Java096_inheritance { public static void main(String[] args) { Second sd = new Second(); sd.prn(); sd.display(); sd.superThisMethod(); } }
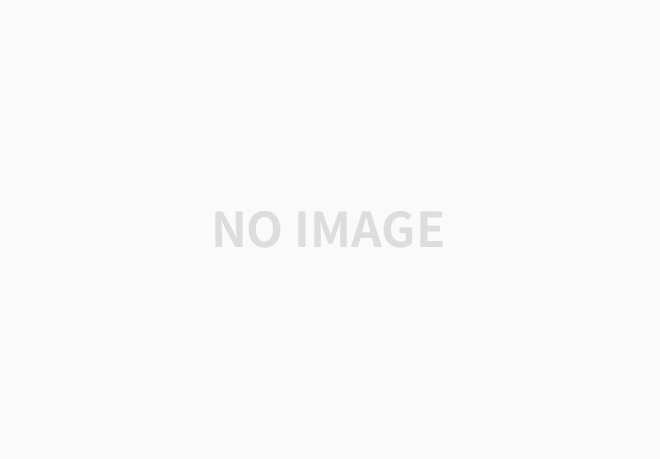
PetOver을 상속받은 DogOver, BirdOver클래스를
main()메소드를 참조하여 아래와 같이 출력이 되도록 구현하시오.
[출력결과]
슈퍼클래스 move() : 애완동물이 움직입니다.
서브클래스move() : 날아갑니다.
Java097_inheritance.java
class PetOver { int age; // 애완동물 개월수 public void move() { System.out.println("수퍼클래스 move():애완동물이 움직입니다."); } } class DogOver extends PetOver { } class BirdOver extends PetOver { @Override public void move() { System.out.println("수퍼클래스 move():새가 날아갑니다."); } } public class Java097_inheritance { public static void main(String[] args) { DogOver dog = new DogOver(); BirdOver bird = new BirdOver(); dog.move(); bird.move(); } }
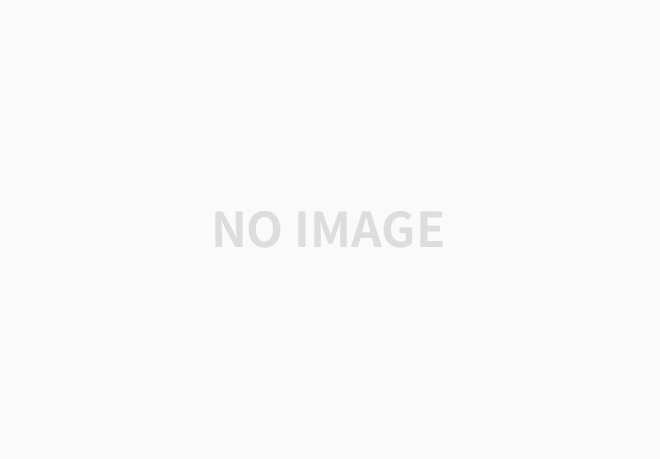
다음과 같은 결과가 나오도록 구현하시오.
(단, main()안의 내용수정과 새로운 Method추가하지마시오)
**** x,y,z에 값채우기***
10을 넘겨받은 생성자
10 20 을 넘겨받은 생성자
10 20 30 을 넘겨받은 생성자
10 20 30
합 : 60
Java098_inheritance.java
class SuperClass { int x, y, z; public SuperClass() { System.out.println("**** x,y,z에 값채우기***"); } public SuperClass(int k) { this(); this.x = k; System.out.println(k + "을 넘겨받은 생성자"); } public SuperClass(int a, int b) { this(a); this.y = b; System.out.println(a + " " + b + " 을 넘겨받은 생성자"); } public SuperClass(int a, int b, int c) { this(a, b); z = c; System.out.println(a + " " + b + " " + c + " 을 넘겨받은 생성자"); } public void display() { System.out.printf("%d %d %d\n", x, y, z); } }// end SuperClass class SubClass extends SuperClass { public SubClass(int a, int b, int c) { // 여기를 구현하세요.////////////////////// super(a,b,c); display(); sumData(); ////////////////////////////// } public void sumData() { System.out.println("합 : " + (x + y + z)); } }// end SubClass public class Java098_inheritance { public static void main(String[] args) { SubClass ss = new SubClass(10, 20, 30); }// end main( ) }// end class
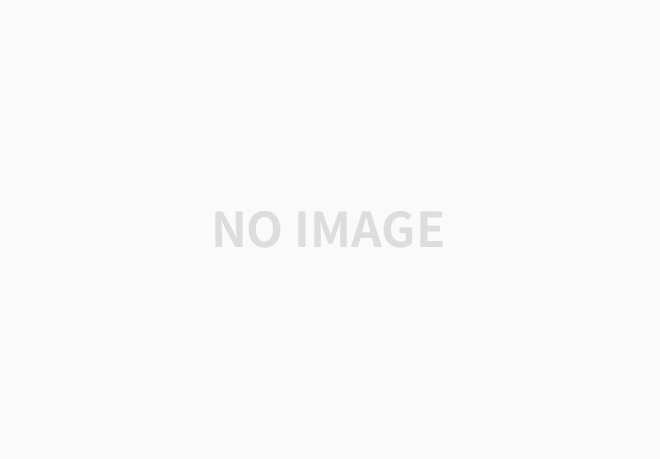
[출력결과]
1000량의 물을 뿌린다
8인승 차량입니다.
Java099_inheritance.java
class Car { private int speed; String color; public void setSpeed(int speed) { this.speed = speed; } }// end class Car///////////////////////// class FireEngine extends Car { private long water; public void setWater(long water) { this.water = water; } void waterSpread() { System.out.println(water + "량의 물을 뿌린다."); } }// end class FireEngine//////////////////// class OwnerEngine extends Car { private int seat; public void setSeat(int seat) { this.seat = seat; } void information() { System.out.println(seat + "인승 차량입니다."); } }// end class OwnerEngine//////////////////// public class Java099_inheritance { public static void main(String[] args) { // 여기를 구현하세요.//////////////////// FireEngine fe = new FireEngine(); fe.setWater(1000); fe.waterSpread(); OwnerEngine oe = new OwnerEngine(); oe.setSeat(8); oe.information(); }//end main( ) }//end class
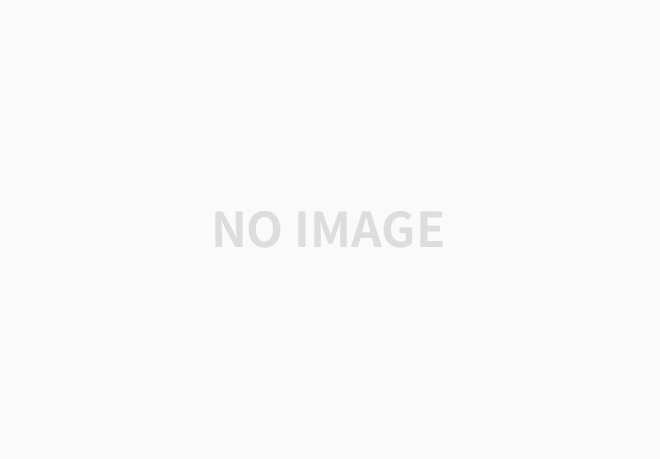
[출력결과]
삼성 SHV-E250S 200000
삼성 SHV-E160S 180000
아이폰 A1586 250000
아이폰 A1524 220000
LG LG-F700L 190000
LG LG-F650L 180000
***************************************************
삼성 SHV-E250S 200000 010-2534-2532 SK
아이폰 A1586 250000 010-2532-5902 LG
LG LG-F650L 180000 010-7280-5283 KT
아이폰 A1524 220000 010-2259-3052 LG
Java100_inheritance.java
class HandPhone { private String maker; // samsung, iphone, lg, saomi private String model; private int price; public HandPhone(String maker, String model, int price) { this.maker = maker; this.model = model; this.price = price; } public String getModel() { return model; } public void setModel(String model) { this.model = model; } public String getMaker() { return maker; } public void setMaker(String maker) { this.maker = maker; } public int getPrice() { return price; } public void setPrice(int price) { this.price = price; } public void display() { System.out.printf("%-4s %-10s %6d\n", maker, model, price); } } class SmartPhone extends HandPhone { private String number; private String type;// 통신타입 kt, lg, sk public SmartPhone(String maker, String model, int price, String number, String type) { super(maker, model, price); this.number = number; this.type = type; } public String getNumber() { return number; } public void setNumber(String number) { this.number = number; } public String getType() { return type; } public void setType(String type) { this.type = type; } @Override public void display() { System.out.printf("%-4s %-10s %6d %s %s\n", getMaker(), getModel(), getPrice(), getNumber(), getType()); } } public class Java100_inheritance { public static void main(String[] args) { HandPhone[] hPhone = new HandPhone[6]; hPhone[0] = new HandPhone("삼성", "SHV-E250S", 200000); hPhone[1] = new HandPhone("삼성", "SHV-E160S", 180000); hPhone[2] = new HandPhone("아이폰", "A1586", 250000); hPhone[3] = new HandPhone("아이폰", "A1524", 220000); hPhone[4] = new HandPhone("LG", "LG-F700L", 190000); hPhone[5] = new HandPhone("LG", "LG-F650L", 180000); for (HandPhone hh : hPhone) hh.display(); System.out.println("***************************************************"); SmartPhone[] sPhone = new SmartPhone[4]; // 여기를 구현하세요.///////////////// sPhone[0] = new SmartPhone("삼성", "SHV-E250S", 200000, "010 - 2534 - 2532", "SK"); sPhone[1] = new SmartPhone("아이폰", "A1586", 250000, "010 - 2532 - 5902", "LG"); sPhone[2] = new SmartPhone("삼성", "LG-F650L", 180000, "010 - 7280 - 5283", "KT"); sPhone[3] = new SmartPhone("아이폰", "A1524", 220000, "010 - 2259 - 3052", "LG"); //////////////////////////////////////// for (SmartPhone ss : sPhone) ss.display(); }// end main() }// end class
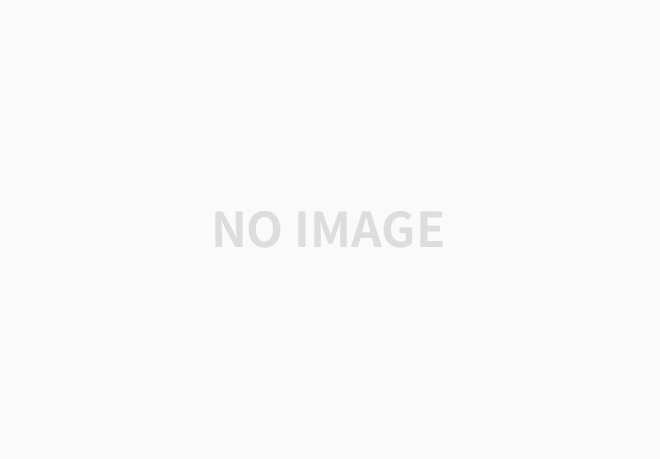
[출력결과]
애완동물이 움직입니다.
애완동물이 움직입니다.
강아지의 이름은 누렁이고, 몸무게는 10Kg입니다.
새의 종류는 앵무새고, 날 수 있습니다.
Java101_inheritance.java
class Pet { int age; // 애완동물 개월수 public void move() { System.out.println("애완동물이 움직입니다."); } }// end Pet class Dog extends Pet { String name; // 강아지 이름 int weight; // 강아지 무게 void sum(int a, int b) { } void sum(int a, int c, int d) { } int getWeight() { return weight; }// end gettWeight( ) }// end Dog class Bird extends Pet { String type; // 새 종류 boolean flightYN; // 날수 있는지 여부 boolean getFlight() { return flightYN; }// end getFlight() }// end Bird public class Java101_inheritance { public static void main(String[] args) { // 출력결과를 참조하여 정상적으로 프로그램 실행이 되도록 여기를 구현하세요. Dog dog=new Dog(); dog.name="누렁이"; dog.weight=10; Bird bird=new Bird(); bird.type="앵무새"; bird.flightYN=true; dog.move(); bird.move(); ////////////////////////////////////////////// // 실행할때 아래 부분의 주석을 해제하세요. System.out.println("강아지의 이름은 " + dog.name + "고, 몸무게는 " + dog.getWeight() + "Kg입니다."); System.out.println("새의 종류는 " + bird.type + "고, 날 수 " + (bird.getFlight() ? "있" : "없") + "습니다"); }// end main() }// end class
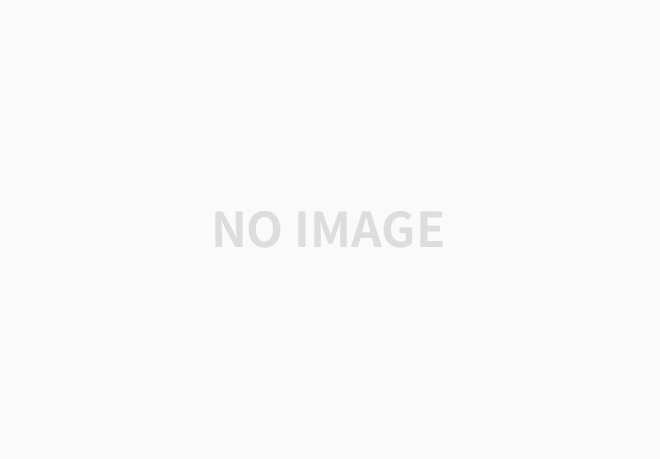
'Back-End > 국비 NCS교과과정' 카테고리의 다른 글
자바 기초 NCS교육과정(20)-캐스팅과 바인딩 (0) | 2019.07.30 |
---|---|
자바 기초 NCS교육과정(19)-상속 문제풀이 (0) | 2019.07.30 |
자바 기초 NCS교육과정(17)-접근제어자 문제풀이 (0) | 2019.07.30 |
자바 기초 NCS교육과정(16)-접근제어자 (0) | 2019.07.30 |
자바 기초 NCS교육과정(15)-클래스 문제풀이 (0) | 2019.07.29 |